1. Overview
In this tutorial, We'll learn how to create an empty Optional instance using Java 8 empty() method. Let us take at the Java 8 Optional empty() example program.
Before that let us take a look at the syntax first and then next its usage.
empty() method is used to create an empty Optional object. That means no value is present for this Optional. Optional is a final class.
2. empty() Syntax
public static <t> Optional<t> empty()
empty is a static method. So this can be invoked directly by its class name as below.
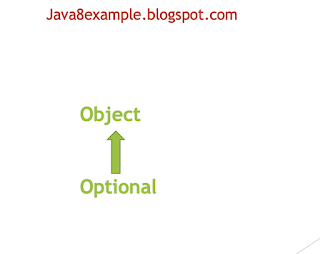
Optional.empty();
This method returns Optional instance.
empty() API Note:
Though it may be tempting to do so, avoid testing if an object is empty by comparing with == against instances returned by Optional.empty(). There is no guarantee that it is a singleton. Instead, use isPresent().
3. Optional empty() Example 1
Let us now write an example of an empty method.
package com.java.w3schools.blog.java8.optional; import java.util.Optional; public class OptionalEmptyExample { public static void main(String[] args) { OptionalemptyOptional = Optional.empty(); System.out.println("Checking optinal value : "+emptyOptional); } }
Output:
In the above program just created an empty Optional instance and printed the returned object. When we print the object directly then its toString() method will be called.
See the below output. It has printed "Optional.empty".
Checking optinal value : Optional.empty
You did not understand. Right? To get a clear idea, let us see its internal implementation.
@Override public String toString() { return value != null ? String.format("Optional[%s]", value) : "Optional.empty"; }
toString() method is overridden Optional class. It is checking if it has value then print it. Otherwise, print return "Optional.empty" String. Because of this, we are seeing a weird output.
Output:
Output:
isPresent() method returns false because it has no value. That's why it did not come inside if block.
In this article, We've seen an example program to create an empty Optional instance using empty() method.
empty() is a static method and will return "Optinal. empty" String when we call toString().
Alway good way to check optinal is empty using isEmpty() method or isPresent().
If we use get() emthod on empty Optinal then will throw runtime exception saying "Exception in thread "main" java.util.NoSuchElementException: No value present".
Output:
All the code is present over GitHub.
4. Another way to check if the created Optional is empty or not
public class OptionalEmptyExample2 { public static void main(String[] args) { OptionalemptyOptional = Optional.empty(); if(emptyOptional.toString().equals("Optional.empty")) { System.out.println("Created Optinal is empty. No value in it."); } } }
Output:
Created Optinal is empty. No value in it.
5. Example to check empty using isEmpty() method
if(emptyOptional.isEmpty()) { System.out.println("emptyOptional is empty"); }
Output:
emptyOptional is empty
Example to check empty using ifPresent():
if(emptyOptional.isPresent()) { System.out.println("checking empty using isPresent() method"); }
isPresent() method returns false because it has no value. That's why it did not come inside if block.
6. Conclusion
In this article, We've seen an example program to create an empty Optional instance using empty() method.
empty() is a static method and will return "Optinal. empty" String when we call toString().
Alway good way to check optinal is empty using isEmpty() method or isPresent().
If we use get() emthod on empty Optinal then will throw runtime exception saying "Exception in thread "main" java.util.NoSuchElementException: No value present".
package com.java.w3schools.blog.java8.optional; import java.util.Optional; public class OptionalEmptyExample3 { public static void main(String[] args) { OptionalemptyOptional = Optional.empty(); if(emptyOptional.isEmpty()) { System.out.println("emptyOptional is empty"); } System.err.println(emptyOptional.isPresent()); System.out.println(emptyOptional.get()); } }
Output:
emptyOptional is empty false Exception in thread "main" java.util.NoSuchElementException: No value present at java.base/java.util.Optional.get(Optional.java:148) at com.java.w3schools.blog.java8.optional.OptionalEmptyExample3.main(OptionalEmptyExample3.java:17)
All the code is present over GitHub.
No comments:
Post a Comment