1. Overview
In this tutorial, We'll learn how to create an Optional object for any value in Java 8. This is a very useful method to create an instance of Optional. Optional is a new class and introduced part of java.util package.
In last tutorial, We've discussed Optional.empty() method.
Let us take a look at Syntax, Example program on of() method.
API note: Returns an Optional describing the given non-null value. If null value, it throws NullPointerException.
2. of() Syntax
public static <T> Optional<T> of(T value)
This is a static method and can be accessed directly with the class name. of() method returns Optional object.
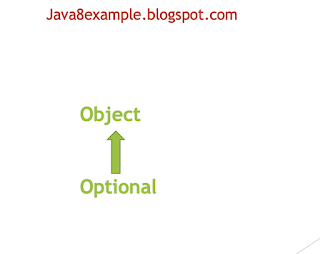
Optional<Integer> Optional.of(10);
3. Java 8 of() Example
Now, let us implement an example program on of() method.package com.java.w3schools.blog.java8.optional; import java.util.Arrays; import java.util.HashSet; import java.util.List; import java.util.Optional; import java.util.Set; /** * java 8 Optional of() method example * * @author venkatesh * */ public class OptionaOfExample { public static void main(String[] args) { // Integer optional Example Optional<Integer> intOptional = Optional.of(10); System.out.println("Integer type optional using of(Integer) method : " + intOptional.get()); // Float optional Example Optional<Float> floatOptional = Optional.of(134.789f); System.out.println("Integer type optional using of(Float) method : " + floatOptional.get()); // String optional Example Optional<String> stringOptional = Optional.of("hello world in java 8"); System.out.println("Integer type optional using of(String) method : " + stringOptional.get()); // List optional Example List<String> names = Arrays.asList("David", "Fernad", "Julia", "Zara", "David"); Optional<List<String>> listOptional = Optional.of(names); System.out.println("Integer type optional using of(List<String>) method : " + listOptional.get()); // Set optional Example Set<String> namesSet = new HashSet<>(names); Optional<Set<String>> setOptional = Optional.of(namesSet); System.out.println("Integer type optional using of(Set<String>) method : " + setOptional.get()); } }
of() method can take any type and returns the same type of Optional. In the above example, Created Optional objects for Integer, Float, List and Set values.
Output:
Integer type optional using of(Integer) method : 10 Integer type optional using of(Float) method : 134.789 Integer type optional using of(String) method : hello world in java 8 Integer type optional using of(List<String>) method : [David, Fernad, Julia, Zara, David] Integer type optional using of(Set<String>) method : [Julia, David, Fernad, Zara]
4. of() Method argument NULL
of(T t) method accepts any value. So, passing null also is a valid case. We will see now what will happen if null is passed.Optional optional = Optional.of(null);
Output:
Exception in thread "main" java.lang.NullPointerException at java.base/java.util.Objects.requireNonNull(Objects.java:221) at java.base/java.util.Optional.<init>(Optional.java:107) at java.base/java.util.Optional.of(Optional.java:120) at com.java.w3schools.blog.java8.optional.OptionaOfExample.main(OptionaOfExample.java:40)
Note: Optional object is not directly created using its Constructor because the constructor is declared as private. See this code is calling Objects.requireNonNull() method.
private Optional(T value) { this.value = Objects.requireNonNull(value); }
Here, NullPointerException is thrown because when it creates an Optional object using a constructor. Its constructor checks for non-null value. The following is its internal code and which will be called for every invocation of of() method.
public static <T> T requireNonNull(T obj) { if (obj == null) throw new NullPointerException(); return obj; }
5. Conclusion
In this article, We've seen how to create an Optional for a given value.
Optional is a Static method and belongs to the class. So, directly of() method can be called with the class name.
Any type of value can be passed to of() method as an argument such as Integer, Double, List, ArrayList, Set, Map, Student. etc. Examples are shown for each type.
And also discussed If a null value is passed then will throw run time exception saying NullPointerException. Understanding with example and internal code.
Hope this article helps. Please share it with your friends if you like.
Leave questions in the comment section. We'll reply as soon as possible.
Full example code on GitHub
API Reference
No comments:
Post a Comment